Solving Security Challenges with Snyk Code and Symbolic AI
February 27, 2025
0 mins readHow good is Symbolic AI as an automated expert system for analyzing code paths and detecting security vulnerabilities? Snyk Code is tested and demonstrates the benefits of rule-based, algorithmic systems.
What is Snyk’s Symbolic AI Security Engine?
Snyk’s SAST engine, which performs static security analysis on codebases, is based on a Symbolic AI system, providing better accuracy and faster execution. It also deeply leverages security researchers' expertise to provide a security expert system with a foundation of vulnerability rules.
These traits of Snyk Code give it a technological superiority over traditional pattern-matching (grep-like regular expression searches) to detect vulnerabilities in your code.
SAST Wins Code Security Challenges
Florin Walter is a security practitioner and penetration tester who has shared security challenges and secure code quiz posts on LinkedIn over the last few months.
I was curious whether Snyk would detect some code vulnerabilities across different languages, so I imported Florin’s code repository. We will review the findings throughout the rest of this write-up.
Open Redirect in a Python Flash Application
Flask is a popular web application framework in Python. The following Python code uses different routes to render a home page and a login page.
from flask import Flask, request, redirect, url_for
import logging
app = Flask(__name__)
logging.basicConfig(level=logging.INFO)
def is_authenticated_user():
# This function checks if the user is authenticated and is omitted for brevity
pass
@app.route('/')
def home():
if not is_authenticated_user():
logging.info('Unauthorized access attempt.')
return redirect(url_for('login'))
redirect_url = request.args.get('redirect_url')
if redirect_url:
logging.info(f'Redirecting to: {redirect_url}')
return redirect(redirect_url)
return 'Welcome to the home page!'
@app.route('/login')
def login():
# Simulated login page
return 'Login Page - User authentication goes here.'
if __name__ == '__main__':
app.run(debug=False)
Unfortunately, an inherent open redirect vulnerability is also baked into allowing users and cross-systems to specify a redirect URL.
As a developer, you might have missed it by having to focus primarily on product-related tasks from the product backlog. Still, Snyk runs a static application security test to analyze this Python code and can detect the open redirect vulnerability:
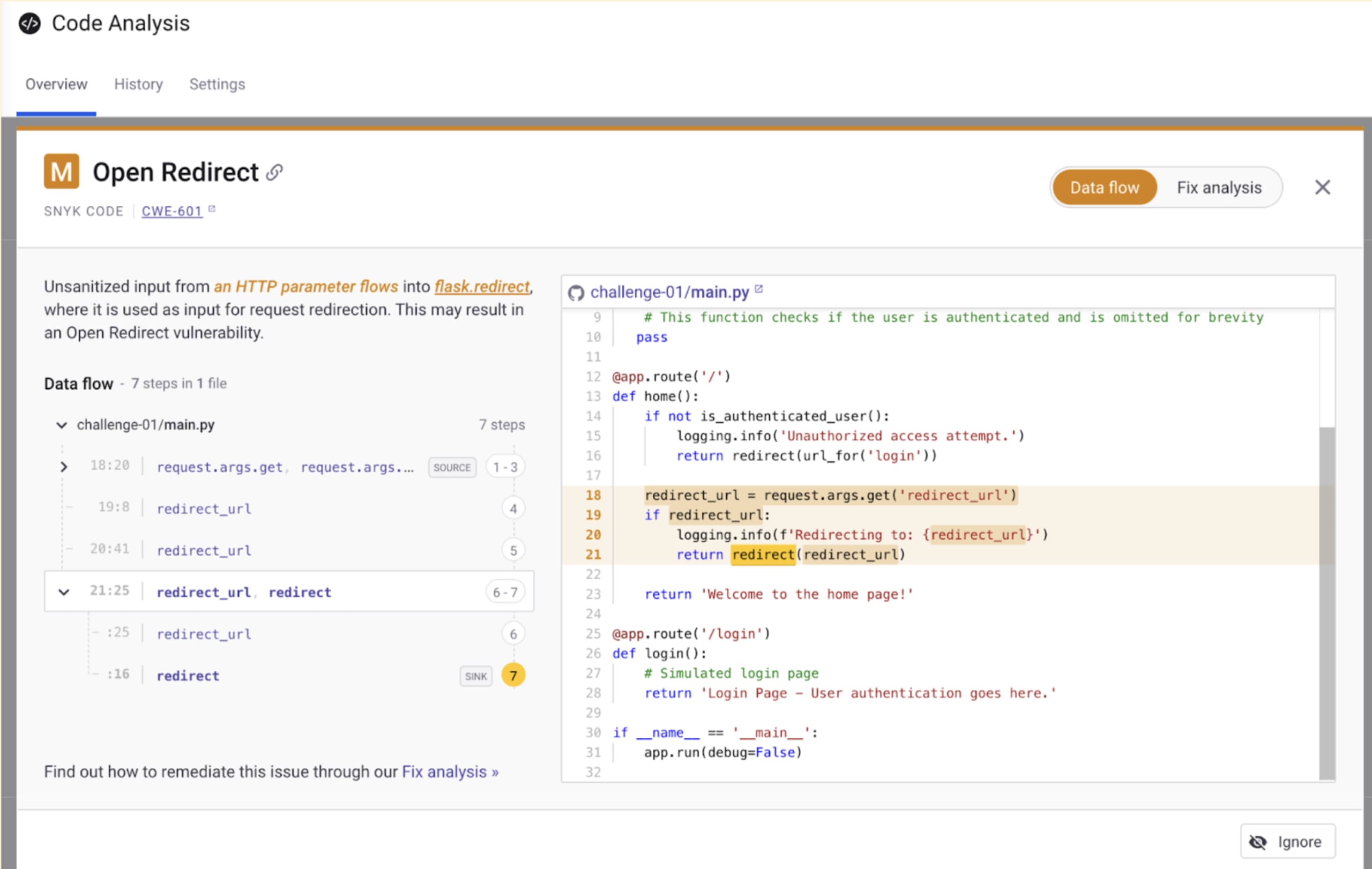
Snyk goes further beyond just detecting insecure code. Thanks to Snyk’s machine learning engine analyzing security defects and their fixes from the open source community, it can deduce and suggest the security fix developers could apply to their code to remediate this open redirect vulnerability.
Following is the screenshot of one out of three of the suggested code fixes that Snyk puts forward, along with best practices for prevention and contextual information about this type of security vulnerability:
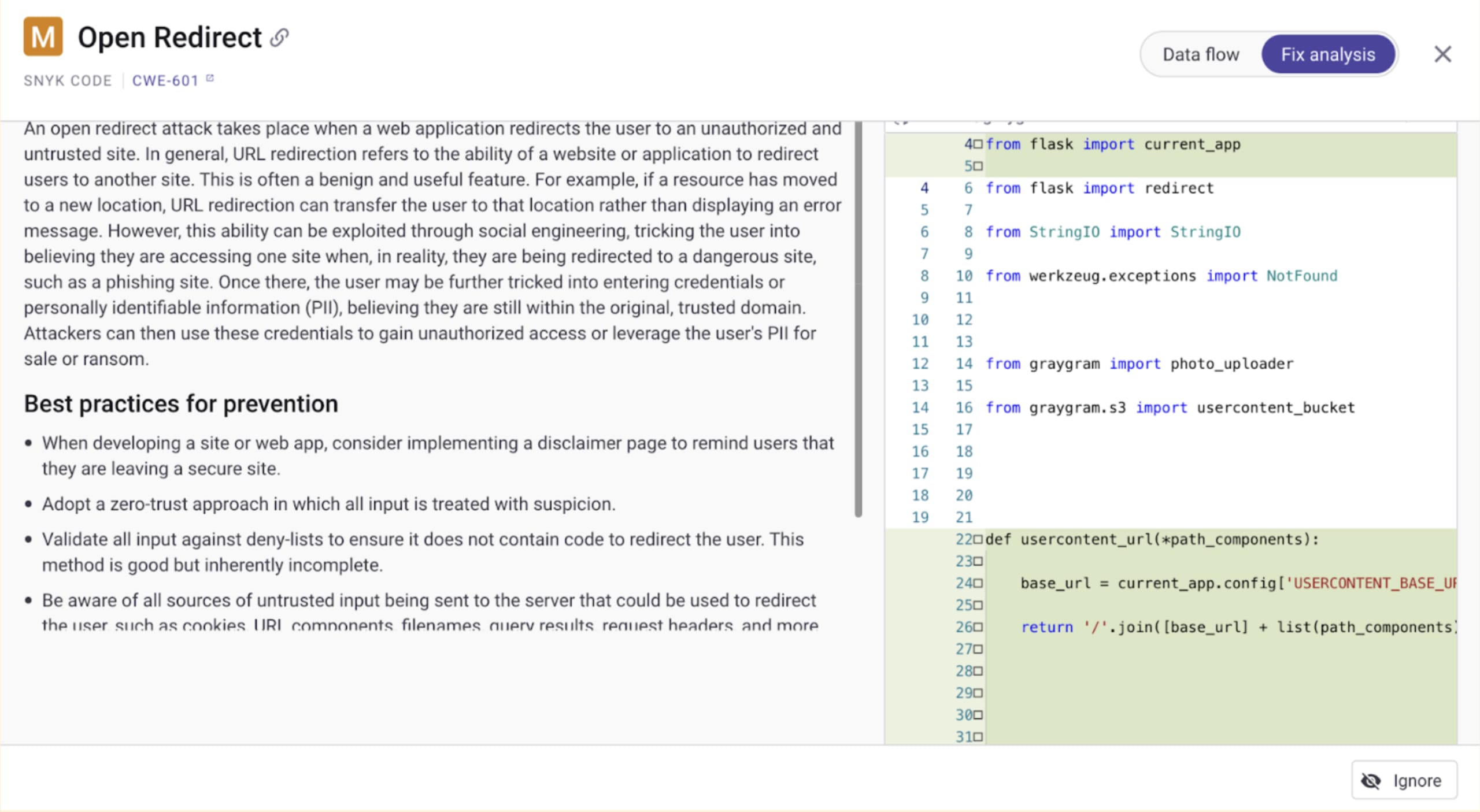
SSRF and XSS in a JavaScript code for a Node.js Application
The next security challenge is written in JavaScript and is based on a server-side Node.js web application built with Express:
const express = require('express');
const axios = require('axios');
const app = express();
app.get('/profile', (req, res) => {
console.log('Received request for /profile');
// Simulated profile data
const profileData = {
name: 'John Doe',
role: 'Developer'
};
res.json(profileData);
console.log('Sent profile data response');
});
app.get('/fetch-data', async (req, res) => {
const url = req.query.url;
console.log(`Received request for /fetch-data with URL: ${url}`);
try {
const response = await axios.get(url);
res.send(response.data);
console.log(`Data fetched and sent for URL: ${url}`);
} catch (error) {
console.error(`Error fetching data from URL: ${url}`, error);
res.status(500).send('Error fetching data');
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Take a second to scan through the code and identify the security vulnerabilities.
I’ve imported this challenge into Snyk, too, and the following are several findings Snyk detected.
Server-Side Request Forgery (SSRF) using Axios
Snyk detected that unsanitized input from an HTTP parameter (req.query.url
) flows into the HTTP client library axios
on line 24. This url
variable specifies the remote URL address to which an HTTP request will be sent.
Because the user can control the full URL, they may abuse it and specify an internal address such as http://localhost and other internal and reserved IP addresses from which they can gather data and extract information.
Snyk identified this insecure code as follows, showing all of the code paths and relevant lines of code that are affected by the vulnerability:
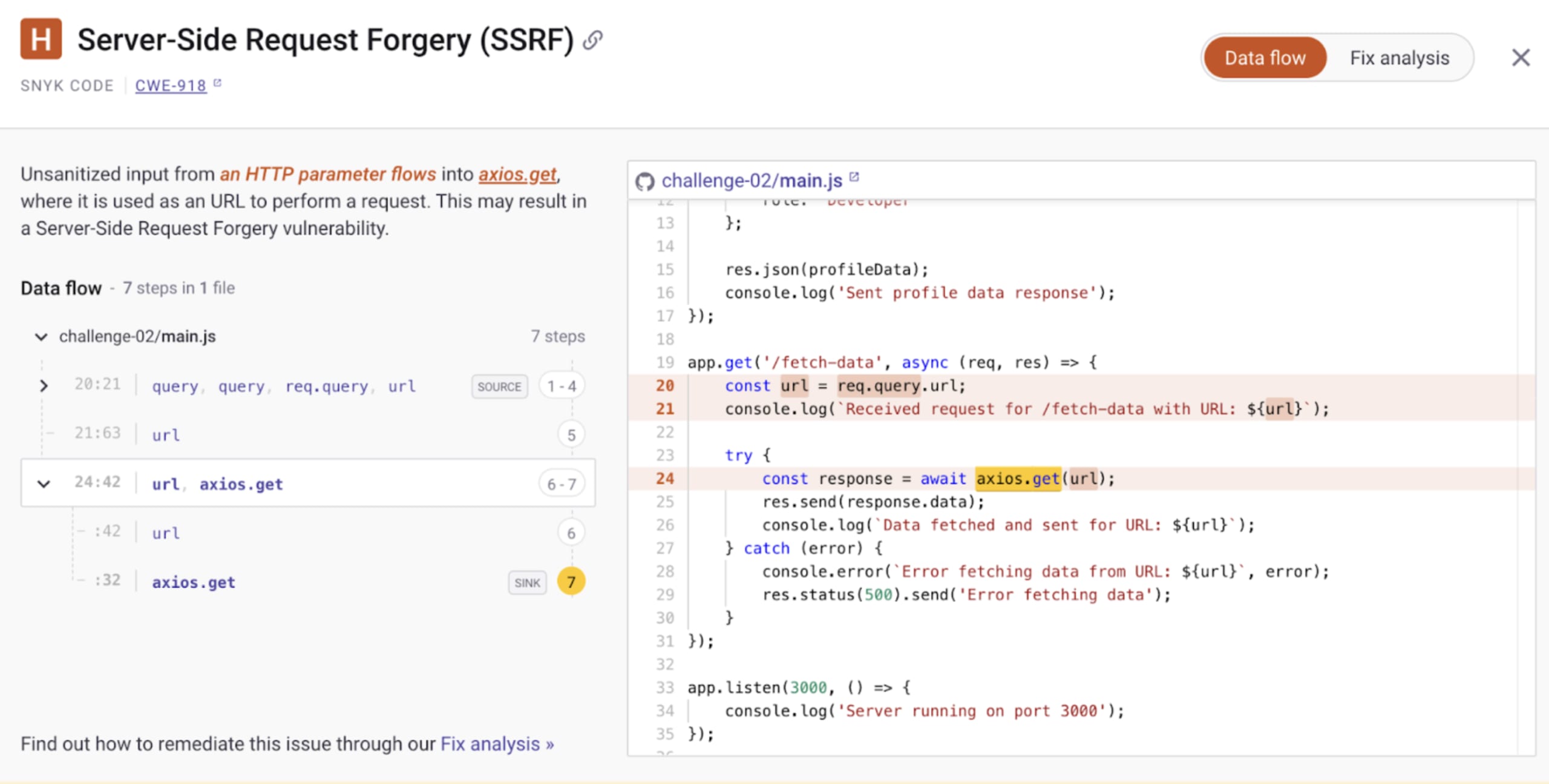
Cross-site Scripting Vulnerability in Express HTTP Response
In continuation to the above code, which is vulnerable to SSRF due to an HTTP request made with a complete URL controlled variable, Snyk detected yet another vulnerability.
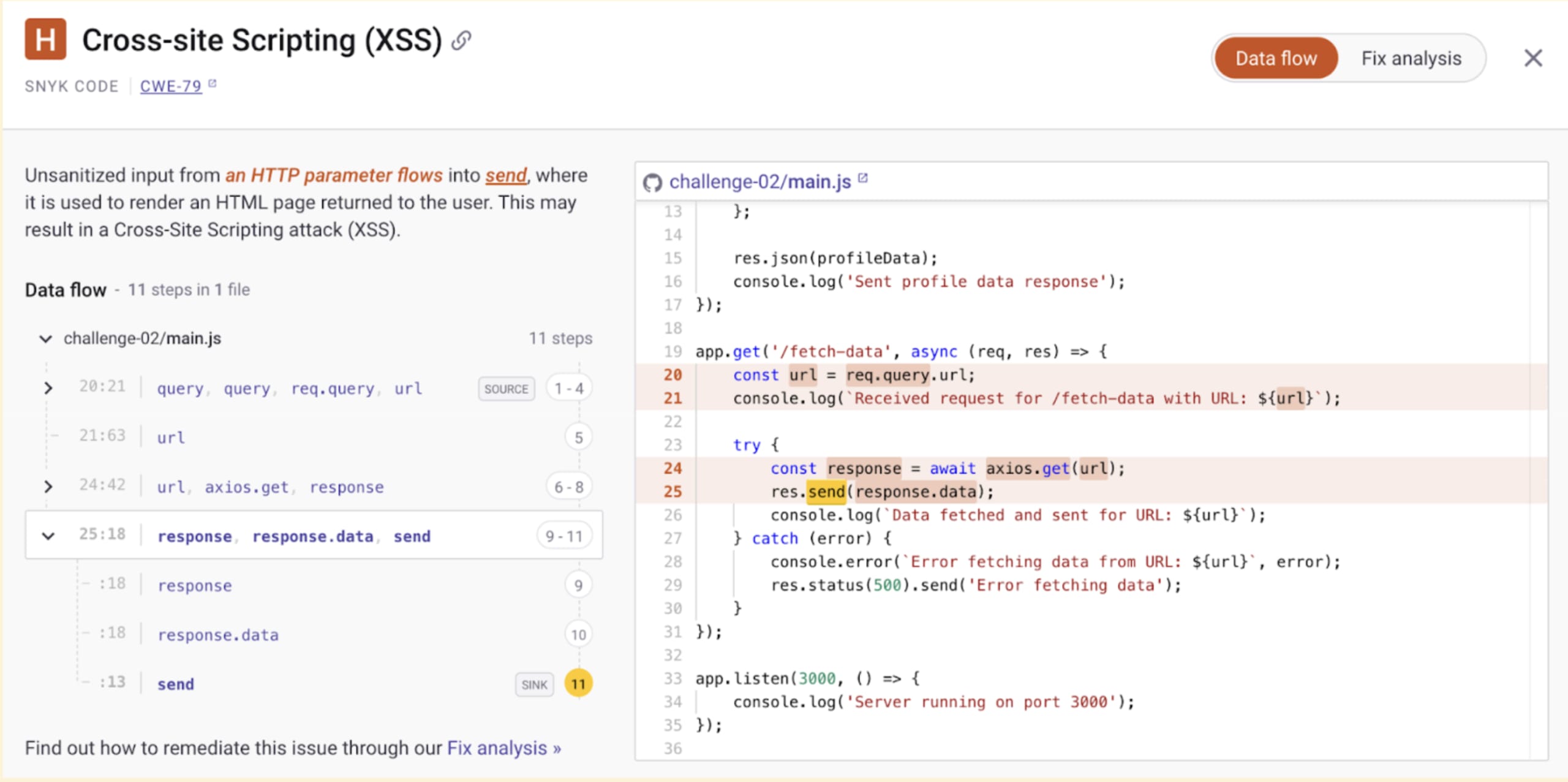
This next one is subtle; it’s not just an SSRF security issue. If you pay close attention to the code highlighted in line 25 by Snyk analysis, it shows that the HTTP response is being sent as-is to the browser:
1res.send(response.data)
By default, Express will set its HTTP response headers to application/html
, which means that if the attacker controls the remote server, they can plant malicious JavaScript code to trigger an XSS on the client side, with the browser interpreting the complete HTML response.
Another insecure code finding that Snyk detects is a potential CRLF injection. Look at this part of the Express web application code from above:
try {
const response = await axios.get(url);
res.send(response.data);
console.log(`Data fetched and sent for URL: ${url}`);
} catch (error) {
console.error(`Error fetching data from URL: ${url}`, error);
res.status(500).send('Error fetching data');
}
Snyk’s SAST easily picks up on this potential CRLF injection as part of the vulnerabilities findings:
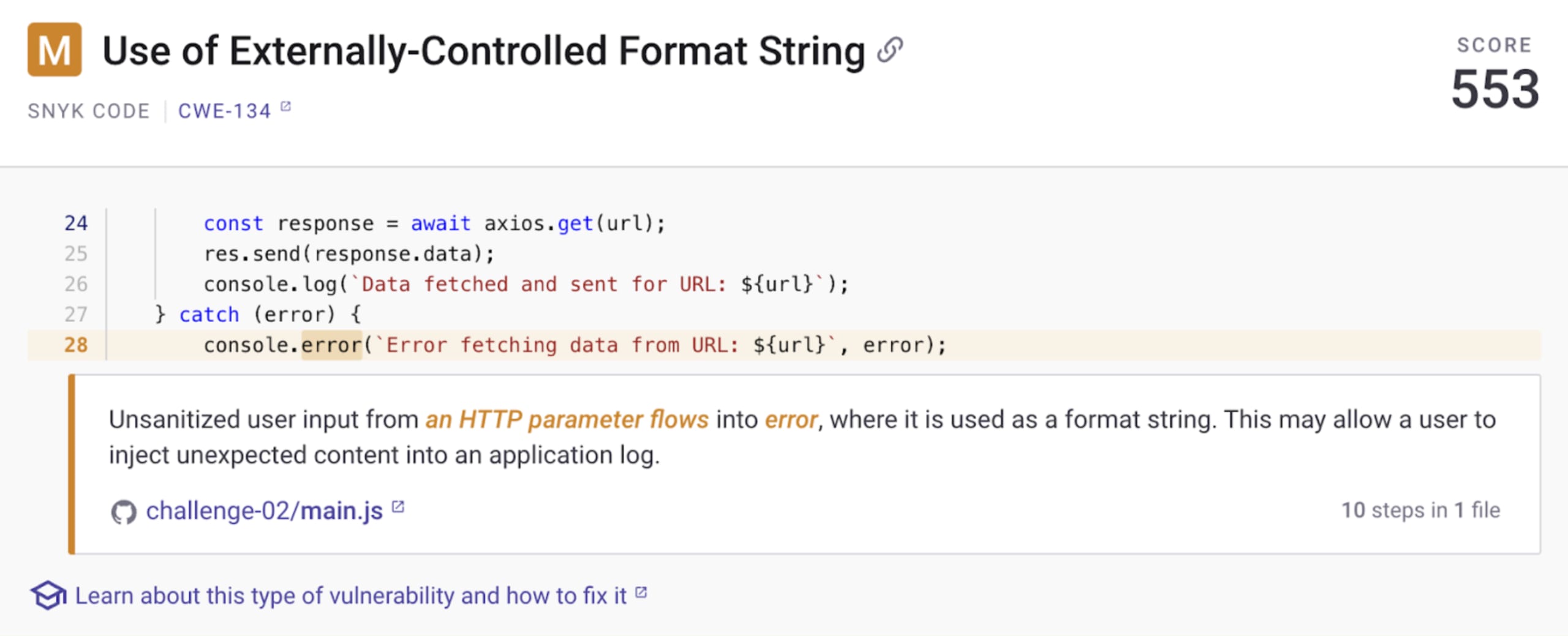
How can Snyk protect my code from security vulnerabilities?
Snyk’s static application security testing tool, known as Snyk Code, helps developers find vulnerabilities in their code in real time by inlining insecure code findings in their IDE.
It’s free to get started - install the VS Code Snyk extension and crush those security bugs!
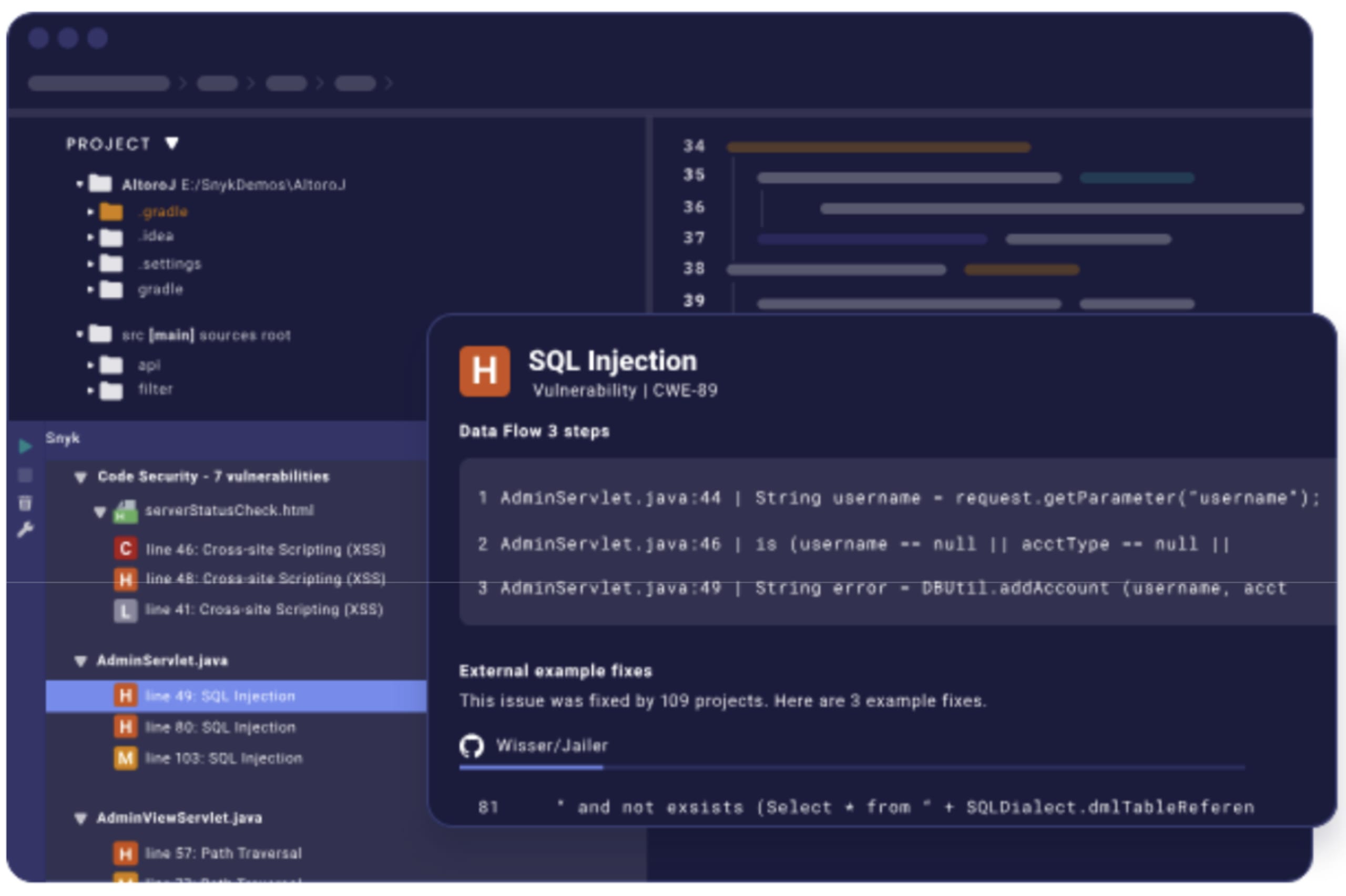
Free online code checker tool
Secure your code before your next commit.